Solving 'TypeError: 'int' object is not callable' in Python
PYTHONOPEN SOURCE
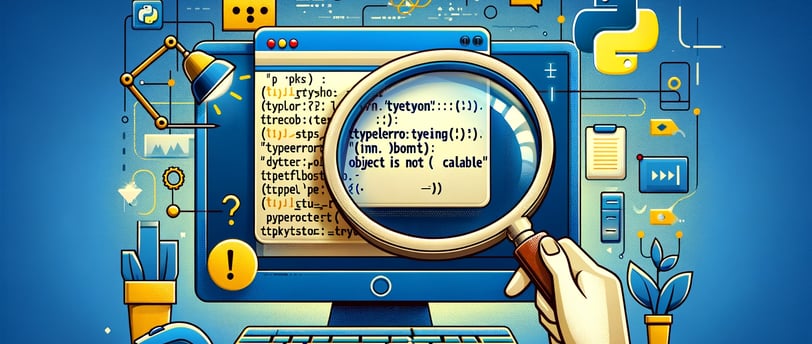
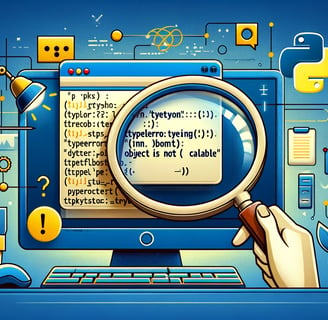
When you program in Python, you will often encounter a variety of error messages. One common type is a "TypeError." This occurs when an operation or function is applied to an object of an inappropriate type. The Python interpreter cannot perform the operation because it's not designed to handle that type in the given context.
One specific TypeError that both new and seasoned developers might run into is the "TypeError: 'int' object is not callable." This blog post will focus on this error. We will explore what this error message means, look at why it happens, and discuss how to fix it. By the end of this post, you should be able to identify and resolve this error in your own code.
Understanding the Error
The error message "TypeError: 'int' object is not callable" tells you a couple of important things. First, the object involved is an integer (int). Second, somewhere in your code, you are trying to use this integer as if it were a function.
In Python, integers are often used to store numeric data. For example, you might use integers to count items, store a person's age, or keep track of scores in a game. These are all typical uses where integers come into play.
The problem arises when you accidentally treat an integer like a function. In Python, functions are callable, meaning you can use parentheses to pass arguments to them. However, integers are not functions and trying to use them like this leads to the aforementioned error.
Common Causes of the Error
There are a few common ways this error can slip into your code. Understanding these can help you avoid them.
Cause 1: Misusing Parentheses
One of the simplest mistakes leading to this error is misusing parentheses with integers. For example, consider you have an integer variable defined as follows:
x = 5
If later in your code you mistakenly use x() with parentheses, Python will throw a TypeError because it thinks you are trying to call x as if it were a function.
Example:
x = 5
result = x() # This will cause TypeError: 'int' object is not callable
In this case, simply removing the parentheses after x will solve the problem. You should review your code to ensure that integers are not being used with parentheses.
Cause 2: Overwriting Built-in Functions
Another frequent cause of this error is when you accidentally overwrite a built-in Python function with an integer. Python has several built-in functions that are always available in your programs, such as len(), max(), min(), and sum(). These functions are callable. If you overwrite any of these (or other built-in functions) with an integer, you'll encounter problems when you try to use the function later.
Example:
sum = 10 # Here 'sum' is a built-in function, but now it's overwritten by an integer.
numbers = [1, 2, 3]
result = sum(numbers) # TypeError: 'int' object is not callable
In the example above, sum was originally a function that adds all numbers in a list or tuple. By assigning sum to 10, it is no longer a function but an integer. When the code tries to use sum(numbers), Python expects sum to be callable and finds that it's an integer, resulting in a TypeError.
To prevent this, always avoid using names of built-in functions for your variables. If you find this error in your code, changing the variable name to something non-conflicting will resolve it.
In the next sections, we'll explore more about how to diagnose these issues quickly and discuss some best practices to prevent such errors from creeping into your code in the first place. Stay tuned!
How to Diagnose the Error
When you encounter a "TypeError: 'int' object is not callable," the first step is to understand where the error is happening. Python provides a traceback when it throws an error. This traceback is very useful. It shows you the file name and line number where the error occurred. It also displays the lines of code involved in the error.
To effectively use a traceback, start from the bottom of the message. This part usually tells you exactly what went wrong and where. The top of the traceback shows where the error ended up, but the bottom points to where it started, which is what you need to fix.
Besides using tracebacks, you can also use Integrated Development Environments (IDEs) and linters. IDEs like PyCharm, VS Code, or even Jupyter notebooks provide syntax highlighting and error checking as you type, which can help you avoid simple mistakes. Linters, like Pylint or Flake8, are tools that analyze your code for errors and potential problems. They can catch a misused integer or an overwritten function before you even run your program.
Solutions and Best Practices
Once you've diagnosed your error, fixing it involves understanding the best practices for coding in Python. Let's go through the solutions and best practices related to our two main causes of the "int not callable" error.
Solution to Cause 1: Proper Variable Usage
To avoid mistakenly adding parentheses to integers, always double-check your variable usage. Parentheses should only follow names of functions or classes. If x is an integer, use x without parentheses. Correct usage ensures that Python does not misinterpret the integer as a function call.
Solution to Cause 2: Avoid Using Reserved Words
Python has many built-in functions and reserved words that should not be used as variable names—like sum, list, and str. Overwriting these can lead to unexpected behaviors. Always choose variable names that are descriptive and do not shadow built-in function names. This not only prevents type errors but also makes your code easier to read and understand.
General Best Practices:
Use Descriptive Variable Names: Instead of short or generic names like x or data, use descriptive names like user_age or total_sum. This makes your code more readable and less prone to errors.
Regularly Review Reserved Words: Python’s reserved words and built-in function names are well-documented. Review them periodically to ensure you’re not accidentally using one as a variable name.
Real-World Example
Let's walk through a real-world scenario to see how this error might occur and how to fix it.
Imagine you are writing a program that calculates the average score from a list of scores. You might start with something like this:
scores = [85, 90, 78, 92]
average = sum(scores) / len(scores)
print(average)
Now, suppose you add more functionality to your program, and without realizing it, you introduce a bug by overwriting sum:
sum = 10 # This is meant to be the sum of some other calculations, mistakenly overwriting the built-in sum function.
average = sum(scores) / len(scores) # This will raise a TypeError: 'int' object is not callable.
To fix this error:
Change the variable name sum to something more descriptive that does not conflict with any built-in function names, like total_sum.
Adjust the rest of your code to use this new variable name.
Here's the corrected code:
total_sum = 10 # Corrected variable name
scores = [85, 90, 78, 92]
average = sum(scores) / len(scores)
print(average)
Conclusion
Understanding and resolving TypeErrors like "int object is not callable" is crucial for writing effective Python code. By following the best practices outlined in this post, you can avoid common pitfalls and improve your debugging skills.
We encourage you to share this post if you found it helpful. Also, if you have any tips, experiences, or questions about TypeErrors or other Python issues, please comment below. We love hearing from our readers and learning from each other’s experiences.